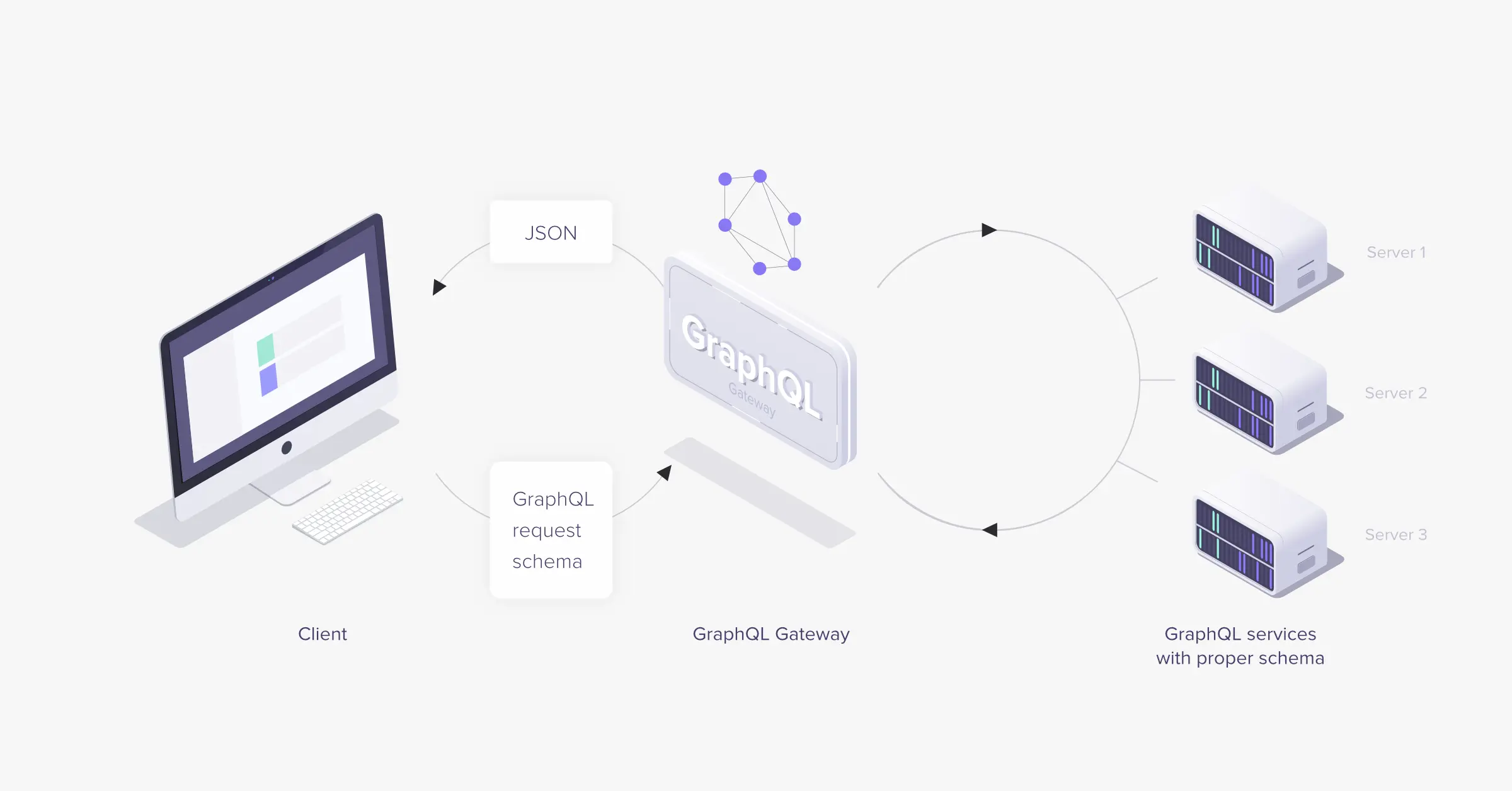
There is probably no better way to learn something in the programming world than to combine making a real app, solving current problems and reading docs.
This is the approach I used when getting to know the basics of GraphQL. I would like to share the results with you, so that you can use this series for educational purposes.
We’re going to build a relatively small and simple app. It will use Yelp API to search for different types of places based on location, search query and category. Yelp provides both REST and GraphQL APIs, which makes it a very good fit for this experiment.
The app also uses Vue 2.6.11, vue-apollo 3.0.3 and vue2-google-maps 0.10.7.
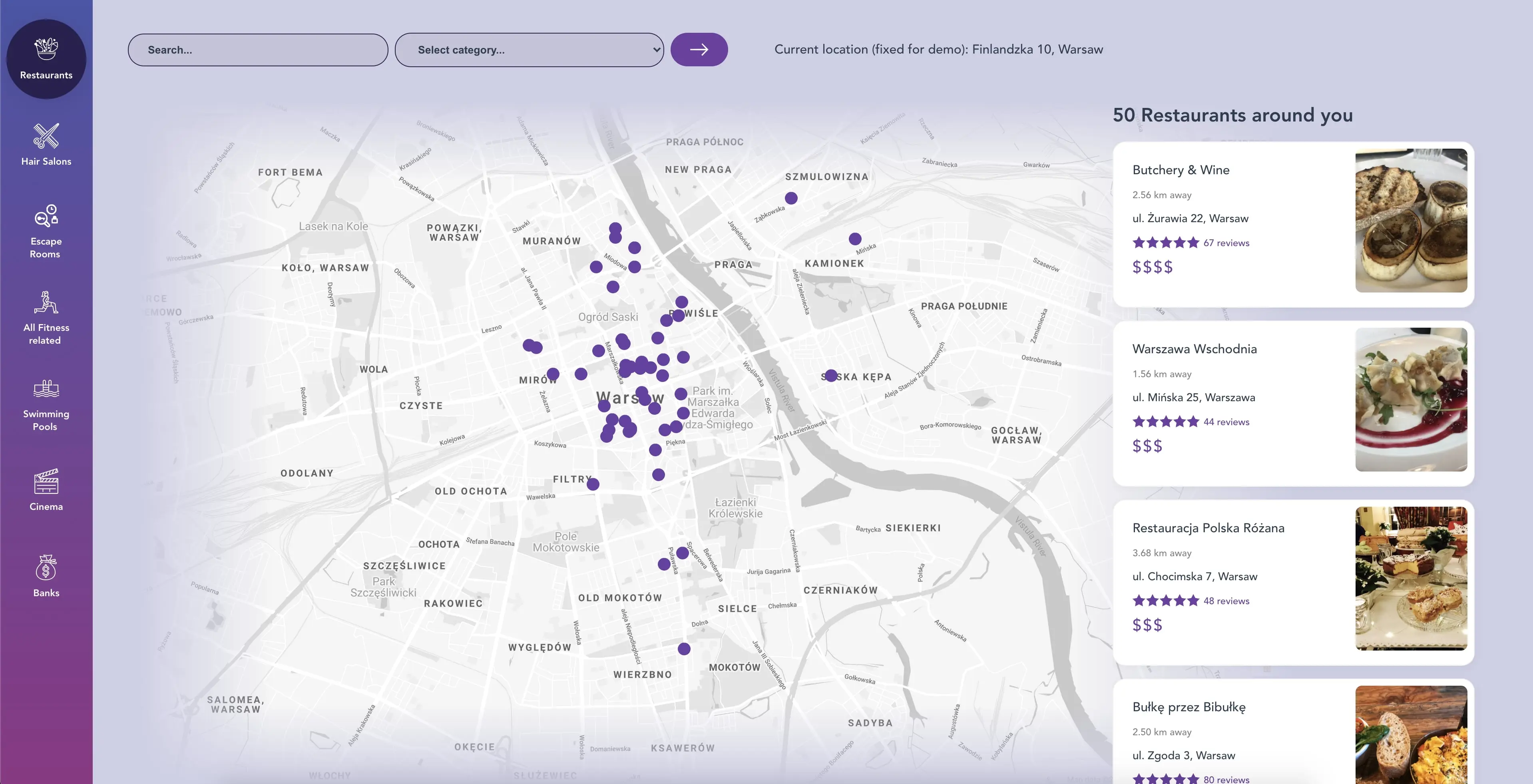
In the first article of the series, let’s learn about GraphQL vs REST approaches to help you first understand both technologies.
Why GraphQL emerged
In recent years, the rapid rise in the popularity of cloud computing services created numerous opportunities to build web and mobile apps that rely on data from APIs.
The more apps there were on the market, the more it became obvious that fetching data needed to be efficient, both for network traffic and user experience. Your app needs to retrieve only the data that it requires to work.
Even though REST can handle under or over-fetching to some extent e.g. through query params handled by backend, GraphQL was created by Facebook as an answer to the data loading problem and has since become a very popular and comprehensive solution.

What is the REST approach in frontend development
REST is an acronym for REpresentational State Transfer. It is an architectural style for distributed hypermedia systems and was first presented by Roy Fielding in 2000 in his famous dissertation.REST uses URLs in most cases to receive or send information.
In summary, the client is stateless and you use HTTP methods for what they are designed for in order to interact with your server resources. You also leverage HTTP status codes, media types, content negotiation.
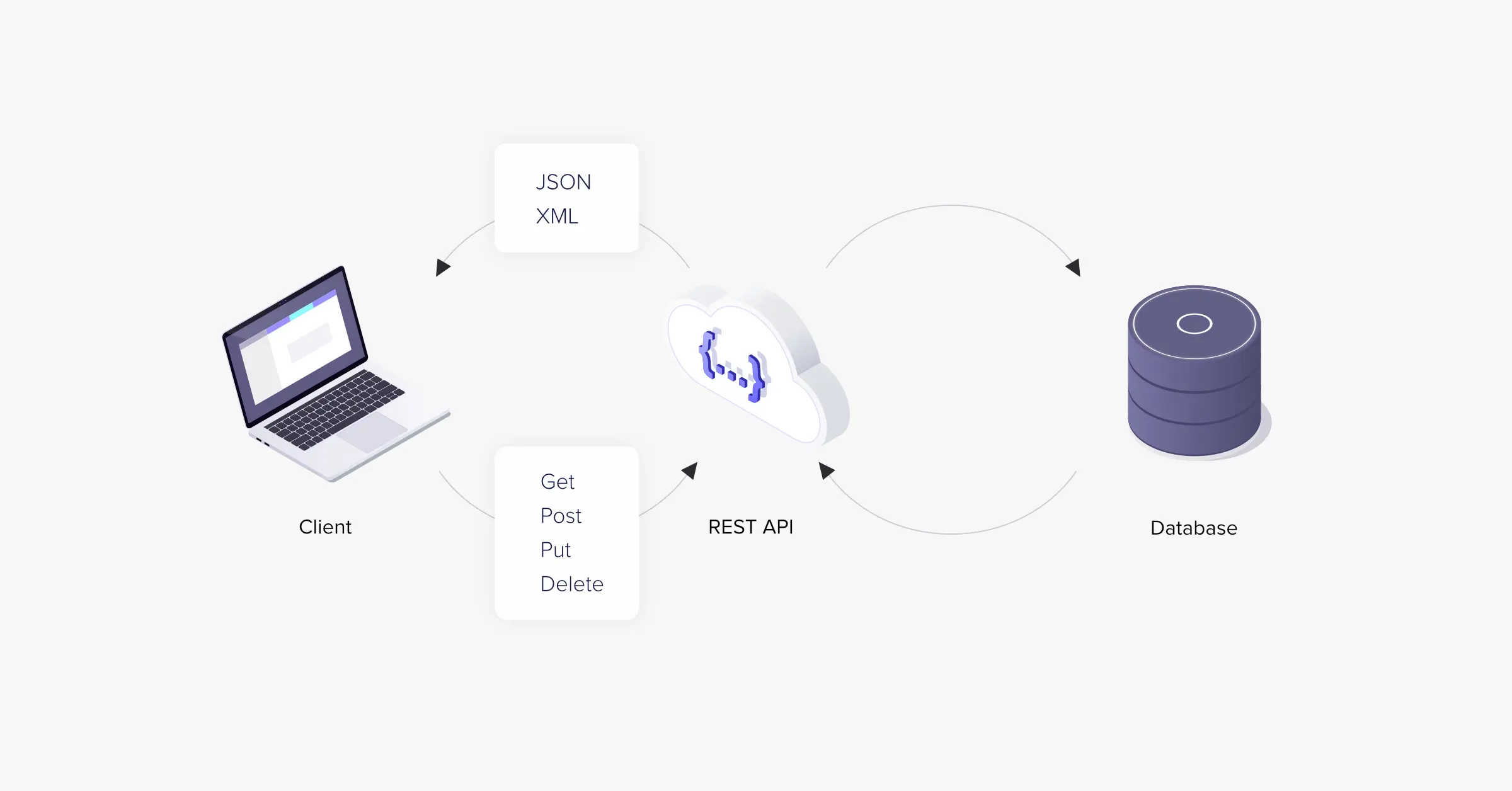
What is the GraphQL approach in frontend development
GraphQL gives frontend developers the ability to design API calls, where the data fetched meets specific requirements. GraphQL resolves the problem of the under- and over-fetching of data.
GraphQL uses SDL (Schema Definition Language). This is something new to learn, but it’s fairly simple. https://graphql.org/learn/schema/
It sometimes happens in development teams that the frontend team has to mock up some data in early sprints. If there is a risk of that, GraphQL helps improve the productivity of the team, because the schema can be defined in the early days of the project. The frontend team can create sample test data, test their application and later easily switch to real API.
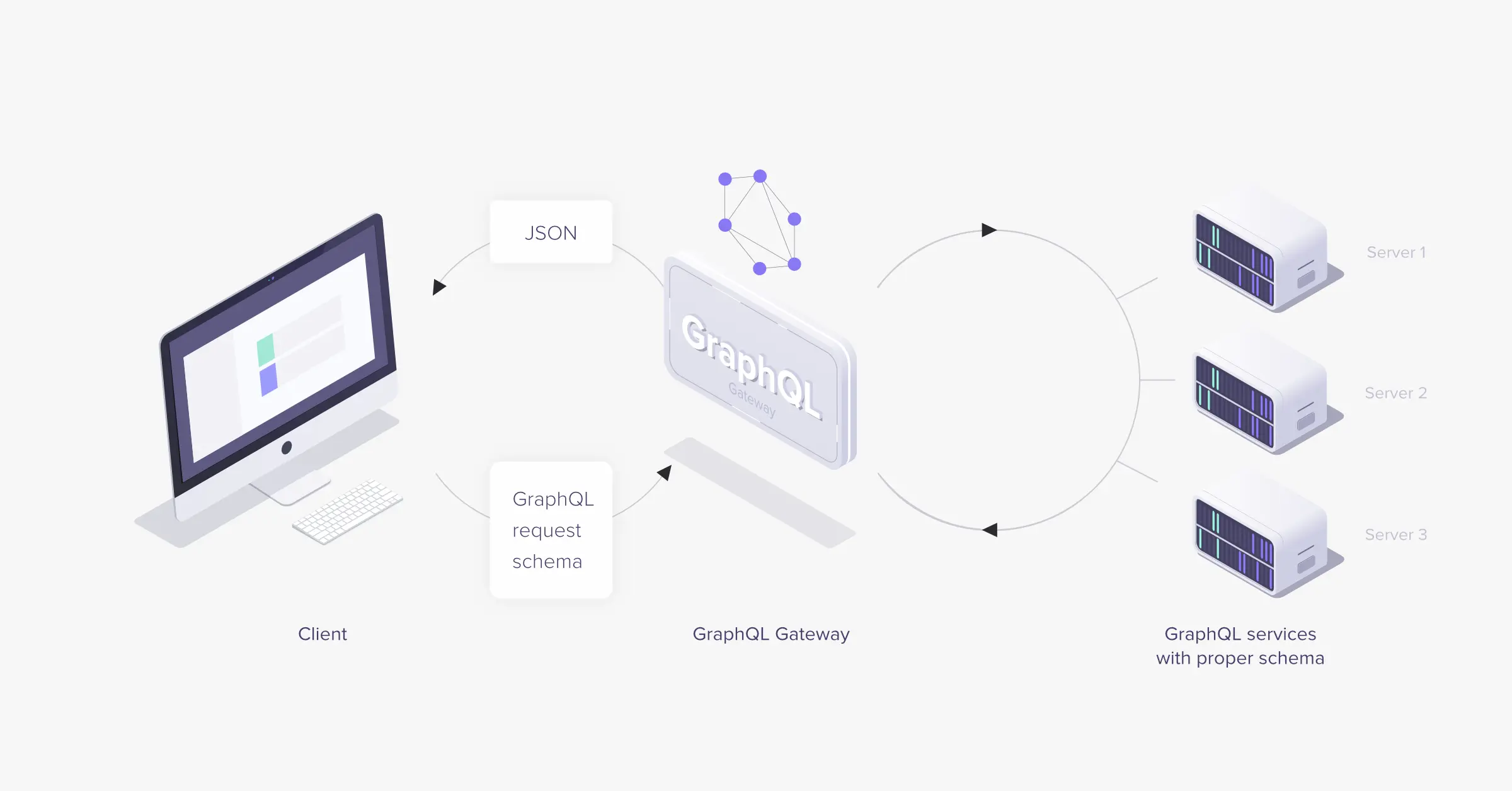
How they differ
In REST, the server determines the shape and size of the resource.
In GraphQL, developers define the queries and types of information they want to download.
In REST, developers call a specific endpoint, which is the identity of an object.
In GraphQL, developers call the main endpoint, but the resources fetched within it are separate from its identity.
This is visible in the example below. In the REST approach, we make 2 requests to different endpoints, whereas in the case of GraphQL, a properly structured query will fetch only the keys needed in one request.
REST:
// HTTP GET request example - 2 requests to get all data needed
// REQUEST
axios.get(`${BASE_URL}/users/${id}`)
// RESPONSE
{
"user": {
"id: "Aks82Jnds10",
"name: "John Doe",
"address": "Sample Address",
"birth_date": "01.01.1990"
}
}
// REQUEST
axios.get(`${BASE_URL}/users/${id}/posts`)
// RESPONSE
{
"posts": [{
"id: "KJs82ns7saasd",
"title: "REST vs GraphQL",
"content": "Lorem ipsum",
"comments": [...]
}]
}
GraphQL:
// Request example - 1 request to get all data needed, smaller requests since we ask for fewer keys
// REQUEST
query {
user(id: "Aks82Jnds10") {
id
name
posts(last: 3) {
id
title
content
}
}
}
// RESPONSE - only required keys, only 3 last posts.
{
"user": {
"id: "Aks82Jnds10",
"name: "John Doe",
"posts": [{
"id: "KJs82ns7saasd",
"title: "REST vs GraphQL",
"content": "Lorem ipsum"
}, ...]
}
}
CONCLUSION
These are the basics to understand both approaches. See part 2, where I go step by step through the code responsible for different features both in REST and GraphQL.